Usage
Initialization
void _INIT ProgramInit(void)
{
Configuration.Manager.LoginMode = UA_MODE_DEFAULT;
brsstrcpy(&Configuration.Manager.VCName[0], &'VGA32');
Configuration.Manager.NumVC = 1;
// Logout delay [s]; Set to 0 to disable automatic logout feature
Configuration.Manager.AutomaticLogoutDelay = 0;
//Add default user - Initialize the default user in UserAccess.var
//If no default user is desired, comment this line out.
Configuration.Users[UA_MAI_USER] = UA_DEFAULTUSER;
}
Cyclic Operation
void _CYCLIC ProgramCyclic(void)
{
//Used to keep track of how many logins have occurred
if(gUserAccessMgr.IN.CMD.Login){
loginCount = loginCount + 1;
}
//add configuration into management structure
brsmemcpy(& gUserAccessMgr.IN.CFG, &Configuration.Manager sizeof(gUserAccessMgr.IN.CFG));
gUserAccessMgr.IN.CFG.pUserList = &Configuration.Users;
gUserAccessMgr.IN.CFG.NumUsers = sizeof(Configuration.Users)/sizeof(Configuration.Users[0]);
// this must be called cyclically for proper access management behavior
UserAccessFn_Cyclic( gUserAccessMgr );
//If anyone below user is logged in, reset the selected user
if( gUserAccessMgr.OUT.STAT.CurrentUser.UserLevel < UA_USRLVL_ADMIN ){}
SelectedIndex = 0;
PageStatus.HMI_STATBIT_VIS = 1;
} else {
PageStatus.HMI_STATBIT_VIS = 0;
)
//If password only, hide the username
if( gUserAccessMgr.IN.CFG.LoginMode = UA_MODE_PASSWORDONLY ){
LoginNameStatus.HMI_STATBIT_VIS = 1;
} else {
LoginNameStatus.HMI_STATBIT_VIS = 0;
}
//Populate a list of usernames to show in a dropdown
for (listIndex = 0; listIndex <= UA_MAI_USER; listIndex++) {
UsersNameList[listindex] = Configuration.Users[listindex].UserName;
}
//Limit the selected user to the ones that exist
if(SelectedIndex > gUserAccessMgr.IN.CFG.NumUsers-1){
SelectedIndex = gUserAccessMgr.IN.CFG.NumUsers-1;
} else if (SelectedIndex < 0 ){
SelectedIndex = 0;
} else {
SelectedIndex = SelectedIndex;
}
//Access the selected user
dUser ACCESS ADR(Configuration.Users[SelectedIndex]);
if (gActiveMode == MODE_MACHINE_MANUAL_JOG){
if(gUserAccessMgr.OUT.STAT.CurrentUser.UserLevel >= Configuration.LevelToUnlockMotorJog){
LockMotorJog = 0;
LockAllAxes = 0;
} else if(gUserAccessMgr.OUT.STAT.CurrentUser.UserLevel >= Configuration.LevelToUnlockJogging){
LockMotorJog = 1;
LockAllAxes = 0;
} else {
LockMotorJog = 1;
LockAllAxes = 1;
}
} else {
LockMotorJog = 1;
LockAllAxes = 1;
}
if (gUserAccessMgr.OUT.STAT.CurrentUser.UserLevel >= Configuration.LevelToUnlockJogging){
gLockAllMotion = 0;
} else {
gLockAllMotion = 1;
//get into Auto mode if not signed in to allow jogging
if (gActiveMode != MODE_MACHINE_AUTO){
gRequestedMode = MODE_MACHINE_AUTO;
}
}
if( LockMotorJog AND gJogData.SelectedMode == JOG_MODE_MOTOR){
gJogData.SelectedMode = JOG_MODE_JOINT;
}
if(!brsmemcmp(&gUserAccessMgr.OUT.STAT.CurrentUser.UserName, &'', sizeof(gUserAccessMgr.OUT.STAT.CurrentUser.UserName)){
gUserAccessMgr.OUT.STAT.CurrentUser.UserName = 'Please Login';
}
}
Loupe UX Modal Example
Screenshot
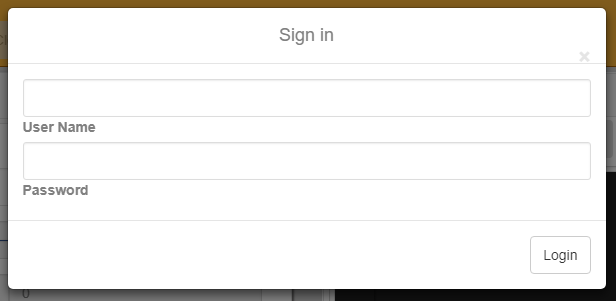
HTML Code Example
<!-- sign in modal -->
<div class="modal fade" id="modalLoginForm" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header text-center">
<h4 class="modal-title w-100 font-weight-bold">Sign in</h4>
<button type="button" class="close lux-btn-set lux-led" data-dismiss="modal" aria-label="Close" data-set-value="1" data-var-name="gUserAccessMgr.IN.CMD.Login">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body mx-3">
<div class="md-form mb-5">
<i class="fas fa-envelope prefix grey-text"></i>
<input type="email" id="defaultForm-email" keyboard="qwerty" type="text" class="form-control validate lux-text-value" data-var-name="gUserAccessMgr.IN.PAR.InputUser.UserName">
<label data-error="wrong" data-success="right" for="defaultForm-email">User Name</label>
</div>
<div class="md-form mb-4">
<i class="fas fa-lock prefix grey-text"></i>
<input type="password" id="defaultForm-pass" keyboard="qwerty" type="text" class="form-control validate lux-text-value" data-var-name="gUserAccessMgr.IN.PAR.InputUser.Password">
<label data-error="wrong" data-success="right" for="defaultForm-pass">Password</label>
</div>
</div>
<div class="modal-footer d-flex justify-content-center">
<button type="button" class="btn btn-default lux-btn-set" data-dismiss="modal" aria-label="Close"
data-var-name="gUserAccessMgr.IN.CMD.Login">Login</button>
</div>
</div>
</div>
</div>